How to create Scalable Applications for a Developer By Gustavo Woltmann
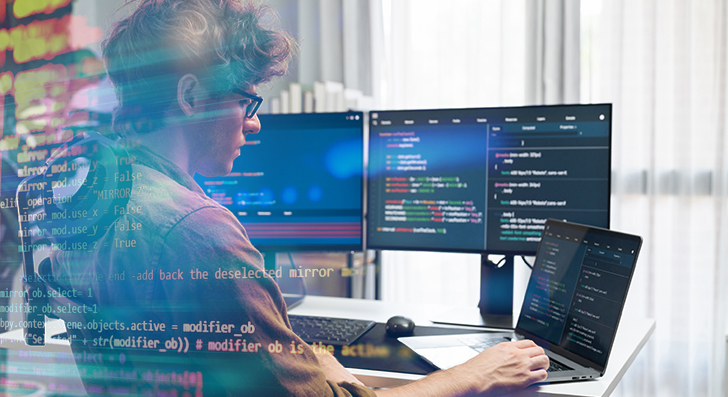
Scalability implies your software can cope with progress—a lot more users, extra knowledge, and a lot more site visitors—devoid of breaking. Like a developer, building with scalability in your mind saves time and worry later on. Here’s a transparent and sensible guideline to help you start out by Gustavo Woltmann.
Design and style for Scalability from the beginning
Scalability isn't really something you bolt on later on—it should be portion of your plan from the start. Many apps are unsuccessful whenever they grow rapidly because the initial design and style can’t tackle the extra load. For a developer, you have to Assume early about how your program will behave stressed.
Start by planning your architecture for being adaptable. Steer clear of monolithic codebases the place everything is tightly connected. As a substitute, use modular design or microservices. These patterns split your application into smaller sized, impartial pieces. Every module or provider can scale By itself without affecting The entire technique.
Also, consider your database from working day just one. Will it need to handle 1,000,000 end users or merely 100? Pick the proper variety—relational or NoSQL—dependant on how your data will develop. Program for sharding, indexing, and backups early, Even though you don’t need to have them still.
A further important level is in order to avoid hardcoding assumptions. Don’t produce code that only will work less than present-day disorders. Contemplate what would materialize if your user base doubled tomorrow. Would your application crash? Would the database slow down?
Use design and style designs that help scaling, like message queues or occasion-driven systems. These support your app manage a lot more requests devoid of finding overloaded.
Any time you Make with scalability in your mind, you are not just planning for achievement—you might be cutting down long run complications. A properly-planned method is easier to take care of, adapt, and improve. It’s superior to arrange early than to rebuild later on.
Use the best Database
Choosing the ideal databases is actually a important Element of making scalable apps. Not all databases are designed precisely the same, and using the Completely wrong one can slow you down or maybe lead to failures as your app grows.
Start out by comprehension your knowledge. Is it really structured, like rows in the table? If Of course, a relational database like PostgreSQL or MySQL is a superb suit. They are solid with relationships, transactions, and consistency. Additionally they assistance scaling approaches like go through replicas, indexing, and partitioning to take care of a lot more traffic and knowledge.
If your knowledge is more adaptable—like user action logs, product catalogs, or paperwork—take into consideration a NoSQL alternative like MongoDB, Cassandra, or DynamoDB. NoSQL databases are improved at handling substantial volumes of unstructured or semi-structured data and may scale horizontally much more quickly.
Also, consider your read through and generate patterns. Do you think you're doing a lot of reads with less writes? Use caching and skim replicas. Are you currently dealing with a hefty publish load? Take a look at databases that may take care of superior write throughput, and even function-dependent data storage methods like Apache Kafka (for non permanent data streams).
It’s also intelligent to Feel forward. You might not will need Highly developed scaling attributes now, but selecting a database that supports them signifies you gained’t need to switch later.
Use indexing to speed up queries. Steer clear of pointless joins. Normalize or denormalize your info dependant upon your entry designs. And constantly watch databases performance as you grow.
In short, the right databases relies on your application’s composition, pace demands, And just how you count on it to improve. Acquire time to choose properly—it’ll preserve plenty of problems later.
Improve Code and Queries
Rapid code is vital to scalability. As your app grows, each modest delay adds up. Improperly prepared code or unoptimized queries can slow down overall performance and overload your system. That’s why it’s important to Establish successful logic from the start.
Begin by crafting clean, very simple code. Prevent repeating logic and remove anything avoidable. Don’t select the most complicated Alternative if an easy 1 works. Maintain your functions brief, concentrated, and simple to check. Use profiling equipment to locate bottlenecks—sites the place your code takes far too extended to operate or employs too much memory.
Next, check out your database queries. These generally slow points down over the code alone. Make certain Each individual query only asks for the information you actually need to have. Stay away from Find *, which fetches almost everything, and instead pick unique fields. Use indexes to speed up lookups. And keep away from doing too many joins, In particular across huge tables.
For those who observe a similar info staying asked for repeatedly, use caching. Keep the effects temporarily making use of instruments like Redis or Memcached this means you don’t need to repeat high-priced functions.
Also, batch your database operations if you can. In lieu of updating a row one by one, update them in groups. This cuts down on overhead and helps make your app additional economical.
Make sure to test with huge datasets. Code and queries that operate high-quality with 100 information may well crash whenever they have to manage one million.
To put it briefly, scalable applications are speedy applications. Keep the code limited, your queries lean, and use caching when needed. These steps assist your application keep clean and responsive, whilst the load will increase.
Leverage Load Balancing and Caching
As your app grows, it's to deal with a lot more consumers and a lot more targeted traffic. If almost everything goes by way of one particular server, it is going to speedily turn into a bottleneck. That’s wherever load balancing and caching can be found in. These two resources assist keep your application rapid, steady, and scalable.
Load balancing spreads incoming site visitors across multiple servers. Instead of a person server accomplishing all the do the job, the load balancer routes people to diverse servers depending on availability. This means no one server will get overloaded. If a single server goes down, the load balancer can send visitors to the Other folks. Resources like Nginx, HAProxy, or cloud-based methods from AWS and Google Cloud make this very easy to build.
Caching is about storing info temporarily so it could be reused swiftly. When users ask for the identical information yet again—like a product web site or possibly a profile—you don’t have to fetch it within the database every time. You can provide it in the cache.
There's two typical different types of caching:
1. Server-facet caching (like Redis or Memcached) retailers details in memory for rapidly obtain.
2. Shopper-side caching (like browser caching or CDN caching) outlets static information near the user.
Caching lessens database load, enhances velocity, and helps make your application much more successful.
Use caching for things that don’t adjust often. And constantly be certain your cache is up to date when facts does alter.
To put it briefly, load balancing and caching are straightforward but impressive tools. Collectively, they assist your app manage additional users, remain rapid, and Get better from issues. If you intend to mature, you'll need the two.
Use Cloud and Container Tools
To construct scalable apps, you would like tools that let your app increase quickly. That’s where cloud platforms and containers come in. They give you versatility, lessen set up time, and make scaling Substantially smoother.
Cloud platforms like Amazon Web Solutions (AWS), Google Cloud Platform (GCP), and Microsoft Azure Enable you to hire servers and expert services as you would like them. You don’t have to purchase hardware or guess long term capability. When site visitors will increase, it is possible to incorporate far more methods with just a couple clicks or routinely employing car-scaling. When targeted visitors drops, you could scale down to economize.
These platforms also give products and services like managed databases, storage, load balancing, and stability instruments. It is possible to target constructing your app rather than managing infrastructure.
Containers are another vital Resource. A container deals your app and everything it needs to run—code, libraries, configurations—into just one unit. This makes it quick to maneuver your app between environments, from a laptop computer towards the cloud, without surprises. Docker is the preferred Device for this.
When your application makes use of numerous containers, applications like Kubernetes allow you to take care of them. Kubernetes handles deployment, scaling, and recovery. If one aspect of one's application crashes, it restarts it routinely.
Containers also allow it to be straightforward to independent parts of your application into solutions. You could update or scale elements independently, which is perfect for performance and dependability.
In short, working with cloud and container resources means you may scale quick, deploy quickly, and recover promptly when issues transpire. If you would like your application to grow without having restrictions, begin working with these tools early. They preserve time, cut down danger, and make it easier to stay focused on constructing, not fixing.
Keep an eye on Everything
Should you don’t watch your software, you won’t know when items go Erroneous. Checking helps you see how your app is doing, location issues early, and make much better selections as your application grows. It’s a vital part of developing scalable check here programs.
Start out by tracking simple metrics like CPU utilization, memory, disk Room, and reaction time. These inform you how your servers and products and services are doing. Resources like Prometheus, Grafana, Datadog, or New Relic will help you acquire and visualize this knowledge.
Don’t just watch your servers—observe your application much too. Regulate how much time it's going to take for users to load pages, how frequently faults happen, and where they occur. Logging equipment like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can assist you see what’s taking place inside your code.
Setup alerts for essential issues. For instance, In case your reaction time goes higher than a Restrict or maybe a provider goes down, you must get notified right away. This aids you repair issues fast, normally in advance of end users even recognize.
Monitoring is usually handy after you make improvements. In case you deploy a fresh function and find out a spike in problems or slowdowns, you are able to roll it again in advance of it triggers real destruction.
As your app grows, visitors and details enhance. With out checking, you’ll overlook indications of difficulties till it’s much too late. But with the best tools in position, you stay on top of things.
In a nutshell, monitoring will help you keep your application reliable and scalable. It’s not almost spotting failures—it’s about comprehension your method and making certain it works properly, even stressed.
Ultimate Views
Scalability isn’t just for major businesses. Even smaller apps need to have a solid foundation. By coming up with cautiously, optimizing correctly, and utilizing the correct instruments, you are able to Create apps that improve effortlessly without having breaking stressed. Get started little, Assume significant, and Construct sensible.